This is part 2 in a multi-part series on iOS unit testing and integration testing. In the last post, we discussed setting up the project and adding some dependencies with CocoaPods.
Today, I’m going to go through setting up some initial code to use the 3rd party libraries to make sure that the libraries are working. Then we’ll set up logic tests and see what breaks with CocoaPods (spoiler: compiler errors ahead!).
SVProgressHUD
First, let’s hook up SVProgressHUD
- Open
ViewController.m
and import SVProgressHUD:#import "SVProgressHUD.h”
- In
viewDidLoad
, create an SVProgressHUD indicator, then dismiss it after 2 seconds:
-(void)viewDidLoad{
[super viewDidLoad];
[SVProgressHUD showWithStatus:@"Running..."];
double delayInSeconds = 2.0;
dispatch_time_t popTime = dispatch_time(DISPATCH_TIME_NOW,
(int64_t) (delayInSeconds * NSEC_PER_SEC));
dispatch_after(popTime, dispatch_get_main_queue(), ^(void) {
[SVProgressHUD dismiss];
});
}
You should be able to run this code now and see a progress indicator with a spinner and the text “Running….”. It should disappear after 2 seconds.
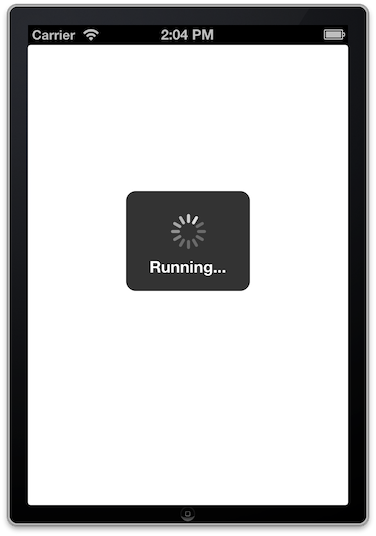
Great! This proves one of our CocoaPods libraries is communicating with the app’s main project and is working properly.
Next we’ll move onto Core Data with Magical Record.